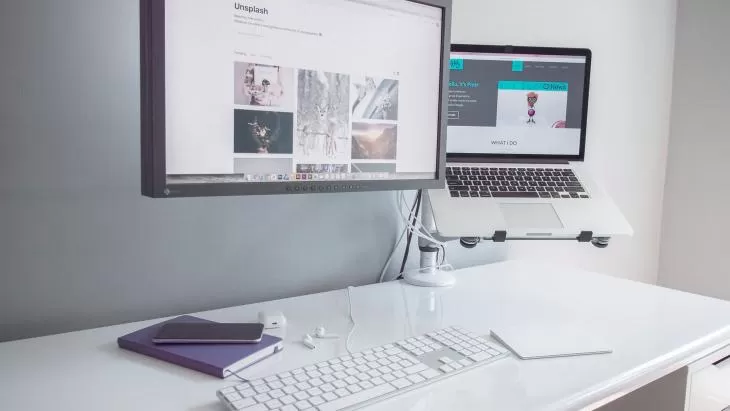
A common business process to automate is moving files from an FTP server. To copy the files from an FTP server we first need to get a file listing. The following routine serves nicely:
function Get-FtpDir ($url,$credentials) { $request = [Net.WebRequest]::Create($url) $request.Method = [System.Net.WebRequestMethods+FTP]::ListDirectory if ($credentials) { $request.Credentials = $credentials } $response = $request.GetResponse() $reader = New-Object IO.StreamReader $response.GetResponseStream() $reader.ReadToEnd() $reader.Close() $response.Close() } |
Let’s set some basic parameters for the file migration:
$url = 'ftp://sftp.SomeDomain.com'; $user = 'UserID'; $pass = 'Password' #single quotes recommended if unusual characters are in use $DeleteSource = $true; #controls whether to delete the source files from the FTP Server after copying $DestLocation = '\DestinationServerAnyLocation'; |
Let’s start the processing with connecting to the FTP server, getting the file list, and processing:
$credentials = new-object System.Net.NetworkCredential($user, $pass) $webclient = New-Object System.Net.WebClient $webclient.Credentials = New-Object System.Net.NetworkCredential($user,$pass) $files=Get-FTPDir $url $credentials $filesArr = $files.Split("`n") Foreach ($file in ($filesArr )){ if ($file.length -gt 0) #this actually happens for last file { $source=$url+$file $dest = $DestLocation + $file $dest = $dest.Trim() # this is actually needed, as trailing blank appears in FTP directory listing $WebClient.DownloadFile($source, $dest) } } |
Let’s now delete the source files, if configured to do so:
$credentials = new-object System.Net.NetworkCredential($user, $pass) $webclient = New-Object System.Net.WebClient $webclient.Credentials = New-Object System.Net.NetworkCredential($user,$pass) $files=Get-FTPDir $url $credentials $filesArr = $files.Split("`n") Foreach ($file in ($filesArr )){ if ($file.length -gt 0) #this actually happens for last file { $source=$url+$file $dest = $DestLocation + $file $dest = $dest.Trim() # this is actually needed, as trailing blank appears in FTP directory listing $WebClient.DownloadFile($source, $dest) } } |
Let’s now delete the source files, if configured to do so:
if ($DeleteSource) { Foreach ($file in ($filesArr )){ if ($file.length -gt 0) #this actually happens for last file { $source=$url+$file $ftprequest = [System.Net.FtpWebRequest]::create($source) $ftprequest.Credentials = New-Object System.Net.NetworkCredential($user,$pass) $ftprequest.Method = [System.Net.WebRequestMethods+Ftp]::DeleteFile $ftprequest.GetResponse() } } } |
That’s it in a nutshell. Just don’t try to move zero length files, and trim the filenames for trailing blanks. Ensure your FTP ports are open, and you are good to go!
Start Your PowerShell Migration Project In A Click
Our technology and wide delivery footprint have created billions of dollars in value for clients globally and are widely recognized by industry professionals and analysts.
Want to talk?
Drop us a line. We are here to answer your questions 24*7.