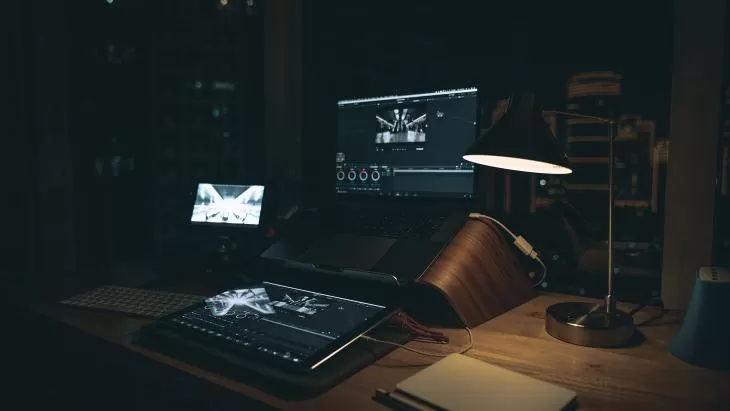
Generating rich text emails with PowerShell
In this post: Generating automatic emails with embedded-reports and link to uploaded csv
we explored sending emails and attachments with HTML tables. Now let’s have a look at alternating row colors in the HTML.
This function will be used later to inject CSS reference to alternating colors into the HTML Table:
Function Set-AlternatingRows { [CmdletBinding()] Param( [Parameter(Mandatory=$True,ValueFromPipeline=$True)] [string]$Line, [Parameter(Mandatory=$True)] [string]$CSSEvenClass, [Parameter(Mandatory=$True)] [string]$CSSOddClass ) Begin { $ClassName = $CSSEvenClass } Process { If ($Line.Contains("<tr>")) { $Line = $Line.Replace("<tr>","<tr class=""$ClassName"">") If ($ClassName -eq $CSSEvenClass) { $ClassName = $CSSOddClass } Else { $ClassName = $CSSEvenClass } } Return $Line } } |
Let’s now define $a as the styles, including the style for alternating rows, which we will use when generating the HTML table:
$a = "<style>" $a = $a + "TABLE{border-width: 1px;border-style: solid;border-color:black;}" $a = $a + "Table{background-color:#EFFFFF;border-collapse: collapse;}" $a = $a + "TH{border-width:1px;padding:5px;border-style:solid;border-color:black;background-color:#DDDDDD}" $a = $a + "TD{border-width:1px;padding-left:5px;padding-right:3px;border-style:solid;border-color:black;}" $a = $a + ".odd { background-color:#ccddee; }" $a = $a + ".even { background-color:#eeeeff; }" $a = $a + "</style>" |
In generating the HTML table, note the reference to the above function in the Pipeline:
$MyOutput = $table | ConvertTo-Html Title, Link, Author, Size, FileName, Modified -head $a -body "<H1>$($rows.count) $($Header)</H1>" | Set-AlternatingRows -CSSEvenClass even -CSSOddClass odd | Convert-HTMLEscape |
As a refresher, we use this function to handle HTML escaping so the HTML comes out delightfully formatted:
Function Convert-HTMLEscape { <# convert < and > to < and > It is assumed that these will be in pairs #> [cmdletbinding()] Param ( [Parameter(Position=0,ValueFromPipeline=$True)] [string[]]$Text ) Process { foreach ($item in $text) { if ($item -match "<") { (($item.Replace("<","<")).Replace(">",">")).Replace(""",'"') } else { #otherwise just write the line to the pipeline $item } } } #close process } #close function |
Want to talk?
Drop us a line. We are here to answer your questions 24*7.